Bird Classifier CNN
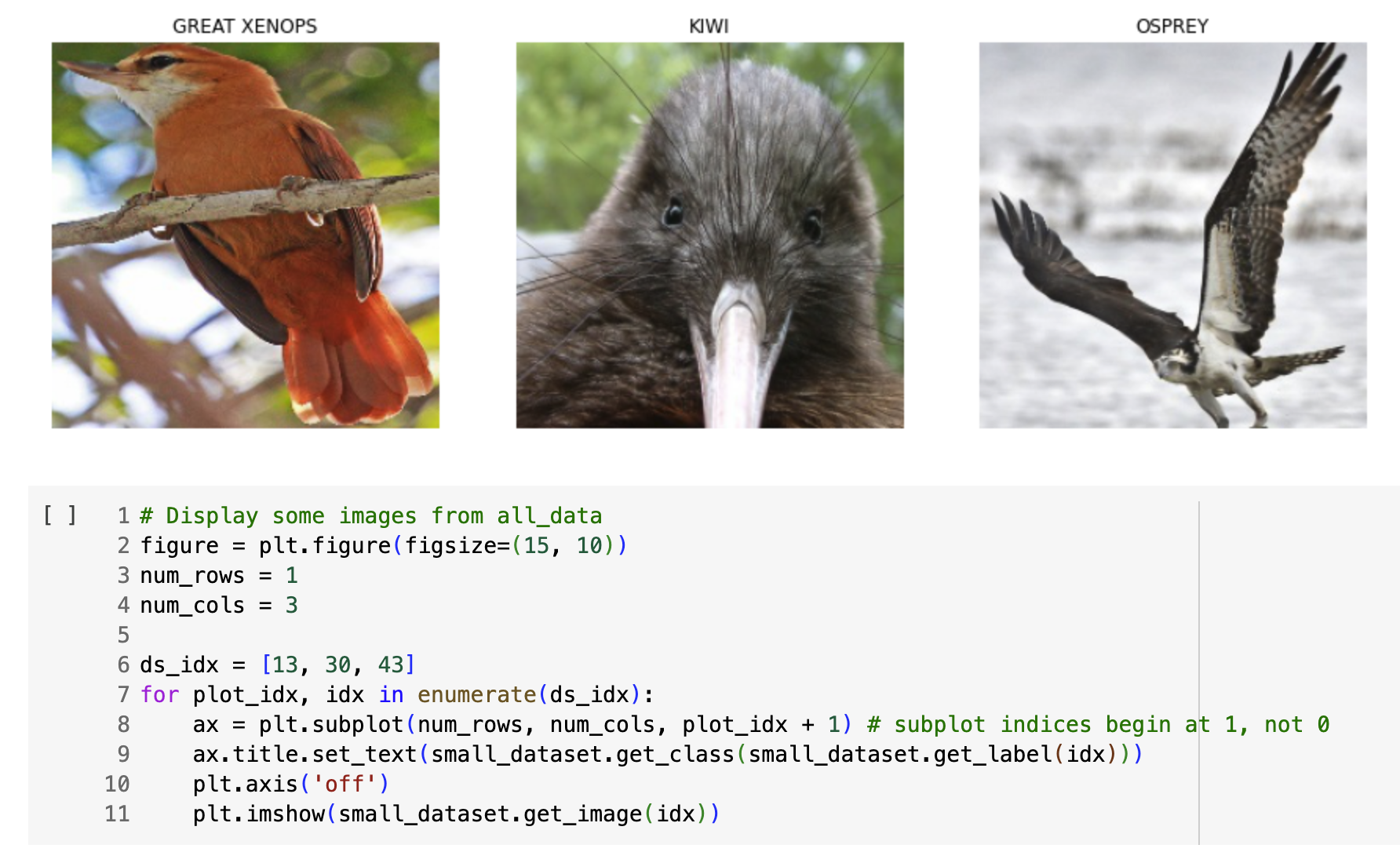
Custom PyTorch CNN with 87% accuracy for bird species classification
Led the development of a custom bird classification model in PyTorch, achieving 87% accuracy on a dataset of 3,113 bird images. Utilized data augmentation and GAN-based image generation techniques to reduce overfitting by 20%, enhancing model generalization. This project demonstrated proficiency in deep learning and image classification, addressing challenges in dataset variability.
Model Architecture
- Architecture Selection:
- Experimented with Variational Autoencoder (VAE) and Generative Adversarial Network (GAN).
- Chose a Convolutional Neural Network (CNN) due to its:
- Superior feature detection capabilities.
- Efficiency in training.
- Proven effectiveness in handling image data.
Sample Code of the Model
class BirdModel(nn.Module):def __init__(self, num_classes=20):
super().__init__()
# input = 224 x 224 x 3
self.conv1 = nn.Conv2d(3, 10, 5)
self.pool1 = nn.MaxPool2d(2, 2)
self.conv2 = nn.Conv2d(10, 20, 5)
self.pool2 = nn.MaxPool2d(2, 2)
self.fc1 = nn.Linear(20 * 53 * 53, 50)
self.fc2 = nn.Linear(50, 20)
def forward(self, x):
self.to(device)
x = self.pool1(nn.functional.relu(self.conv1(x)))
x = self.pool2(nn.functional.relu(self.conv2(x)))
x = x.reshape(-1, 20 * 53 * 53)
x = self.fc1(x)
x = self.fc2(x)
return x
Training Process
- Data Augmentation Techniques:
- Implemented:
- Image rotation.
- Color fixation changes.
- Random erasing.
- Horizontal and vertical flips.
- Designed to simulate diverse real-world scenarios and improve model robustness.
- Hyperparameter Tuning:
- Extensive tuning led to a 15% improvement in model precision and recall.
- Loss Functions:
- Optimized loss functions tailored for classification tasks to enhance performance.